1. Introduction to PyMongo and MongoDB failover testing
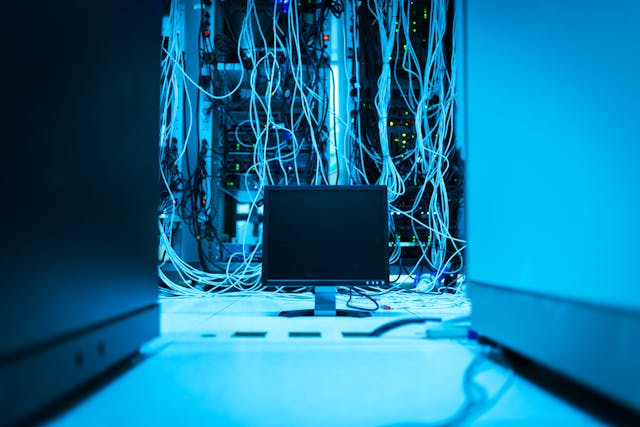
We will examine PyMongo, the Python driver for MongoDB, and how to test MongoDB failover in Python applications in this article. PyMongo offers a potent approach to utilize MongoDB's features in your projects by streamlining interaction with MongoDB databases within Python scripts.
Making sure that distributed systems, such as MongoDB clusters, are resilient to faults is essential when working with them. With failover testing, you can run through several failure scenarios and see how your application responds when a node fails or changes roles.
You may strengthen the resilience of your applications and get them ready for situations when failure is not a question of if, but when, by learning how to test MongoDB failover with PyMongo. Let's delve into the specifics of configuring PyMongo for failover testing in your Python application. 📙
2. Setting up a MongoDB replica set for failover testing
Testing failover situations in your Python program requires setting up a MongoDB replica set. In MongoDB, a replica set is made up of several nodes, with one serving as the main and the rest as secondaries. Make sure MongoDB is installed on your computer before continuing. Next, make a directory with distinct ports for the data of each member. Start by running "mongod --port 27017 --dbpath /path/to/data/node1" to initialize the main node. Next, add two more nodes by using comparable instructions, but with distinct data pathways and ports.
Launch the MongoDB shell after configuring the nodes, then use "rs.initiate()" to configure the replica set. Use "rs.add('localhost:27018')" and "rs.add('localhost:27019')" to add the secondary nodes to the replica set. Use the shell to run "rs.status()" to confirm the configuration. You can now prepare your MongoDB replica set for failover testing. By manually disconnecting or halting the main node, you can replicate failures.
To make sure your Python program can manage unforeseen occurrences like node failures inside your database infrastructure gracefully, it is imperative to test failover scenarios. You may proactively handle possible problems that might occur in a production environment by configuring a MongoDB replica set and modeling different failure situations. Watch this space for our upcoming piece on utilizing PyMongo to provide failover management to your Python application!
3. Installing PyMongo and configuring it for the Python app
To install PyMongo for your Python app, you can use pip, Python's package installer. Open your terminal and run the following command: ```bash
pip install pymongo
```
This command will download and install the latest version of PyMongo on your system. Once installed, you can start configuring PyMongo for your application.
You must establish a connection with multiple MongoDB instances in order to configure PyMongo to manage failover in MongoDB. PyMongo can now automatically switch servers in the event that one goes down thanks to this. This is an example of code that demonstrates how to accomplish this: ✍️
```python
from pymongo import MongoClient
from pymongo.errors import AutoReconnect
client = MongoClient("mongodb://server1:27017,server2:27017/?replicaSet=myReplicaSet")
def connect_with_retry():
while True:
try:
# Attempt to connect to MongoDB
client.admin.command('ismaster')
print("Connected to MongoDB!")
break
except AutoReconnect as e:
print(f"Error connecting to MongoDB: {e}")
```
The addresses of the primary and secondary MongoDB servers are created into an instance of `MongoClient` in this code snippet and placed in a replica set called "myReplicaSet." The `connect_with_retry` method manages any `AutoReconnect` problems that can arise in failover scenarios while attempting to establish a connection to MongoDB.
Using PyMongo with multiple MongoDB instances in a replica set makes sure that failover scenarios may be handled by your Python application automatically and without assistance from you. When your application interacts with MongoDB databases, this setup improves its availability and dependability.
Next, we will discuss how to implement error handling and automatic retries in your Python app using PyMongo to ensure seamless operation during failover events.
4. Writing test cases to simulate MongoDB failover scenarios
It is imperative that you test failover scenarios in your Python application that communicates with MongoDB in order to guarantee system resilience. This section of the PyMongo tutorial series will concentrate on creating test cases that replicate different MongoDB failure scenarios.
When the primary MongoDB server fails, one of the secondary servers takes over as the new primary. This is a common failover situation. You can build a test case that purposefully disconnects from the primary server and verifies that your application can switch to the new primary without experiencing any downtime or data loss in order to test this scenario.
Network partitioning, in which some servers in the cluster are isolated from one another, is another crucial failover situation. You may confirm the operation of your application's connection pooling and failover capabilities under such conditions by building test cases that mimic network partitions inside your cluster.
It is crucial to test for split-brain scenarios, in which distinct regions of the cluster think they are still connected to one another even after being divided. It can be helpful to write test cases that simulate split-brain scenarios to verify how well your program manages such intricate failover events.
In summary, you can make sure that your system is reliable and durable even in the worst of situations by utilizing PyMongo to create thorough test cases that replicate MongoDB failover scenarios in your Python application. By taking a proactive approach to testing, you can find any potential flaws in your application's failover capabilities and fix them before they have a detrimental effect on the user experience.
5. Implementing automatic failover handling in the Python app using PyMongo
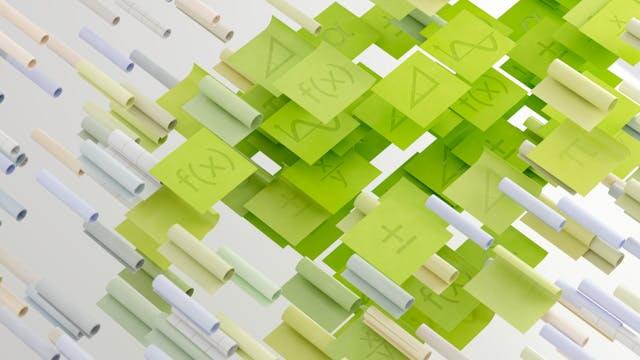
When working with MongoDB clusters, you must incorporate automatic failover handling into your Python program using PyMongo in order to guarantee the robustness and dependability of your service. PyMongo has the ability to recognize and react to modifications in the cluster setup, allowing for automatic failover without the need for human involvement.
You can use PyMongo's built-in functionality, which include monitoring cluster events, preserving server selection preferences, and gracefully resolving network problems, to perform automatic failover management. Your Python program may dynamically adjust to modifications in the MongoDB cluster topology by appropriately configuring PyMongo, guaranteeing uninterrupted operation even in the event of node failures or primary elections.
Establishing a strong retry strategy for database operations is a crucial part of putting automatic failover handling into practice. With PyMongo, you may create unique error-handling procedures that can attempt unsuccessful operations again on various cluster nodes until they are successful. This method reduces interruptions brought on by temporary failures or cluster reconfigurations and helps to preserve data consistency.
By taking advantage of PyMongo's read and write concerns support, you may manage the data routing throughout the MongoDB cluster in case of failover. You may make sure that queries are sent to the best nodes based on their role and availability in the replica set or sharded cluster by including proper read preferences and write concerns in your code.
Using PyMongo's logging features, it is essential to monitor and log cluster events in addition to managing failover scenarios. You may maximize performance under different scenarios and fine-tune your automatic failover techniques by knowing how your application responds to changes in the MongoDB architecture.
You may create reliable Python programs that function well in dynamic MongoDB environments by adhering to best practices and making use of PyMongo's sophisticated features for automatic failover handling. It is important to extensively test these implementations to make sure that your application can handle errors gracefully and continue to provide high availability for users engaging with your database-backed services.
6. Tips for monitoring and optimizing MongoDB failover performance
Ensuring the stability and dependability of your Python program depends on tracking and refining MongoDB failover performance. The following advice can help you handle this issue more skillfully:
1. **Set up comprehensive monitoring**: Use tools like as DataDog or MongoDB Cloud Manager to keep an eye on important metrics including server statistics, replica set status, and replication lag. To be informed of any problems that can impact failover performance, set up alerts.
2. **Review logs on a regular basis**: MongoDB log analysis can reveal important information about the state of your database cluster. Watch out for faults or alerts about elections, replication, or network problems that can affect failover.
3. **Test failover scenarios**: Make sure your application can effectively handle node failures without compromising user experience by regularly carrying out failover tests. To test how well your system responds to server failures, use tools such as Chaos Monkey.
4. **Optimize network configuration**: Make sure your network configurations—such as TCP keepalive settings or high availability network interface configurations—are optimal for MongoDB's needs.
5. **Tune replica set priorities**: In failover scenarios, members' consideration for primary status can be influenced by modifying the replica set priorities. Think about allocating priorities according to the demands of workload and server capabilities.
6. **Monitor resource utilization**: Monitor the amount of memory, CPU, and disk space used by each MongoDB node in order to spot any possible bottlenecks that can affect the performance of failover. Adjust resource levels as necessary to keep system performance at its best.✉️
These pointers will help you make your Python application more resilient to unplanned node failures and other disruptions in your database cluster. You can also continuously analyze and optimize your MongoDB failover performance.
7. Best practices for ensuring data consistency during failover events
When utilizing MongoDB, ensuring data consistency during failover events is essential to preserving the integrity of your application. To assist you in efficiently managing data consistency, consider the following best practices:
Enable appropriate error handling: Make sure to identify and manage any exceptions that might arise during failover situations. By doing this, data loss or corruption during database transitions between primary and secondary nodes will be less likely. 😡
2. **Use write concern**: Determine the proper write concerns in accordance with the specifications of your application. You may make sure that data is correctly replicated across nodes before moving further by indicating the level of acknowledgment that MongoDB must provide following a write transaction.
3. **Use read preferences**: Set up read preferences to control how members of the replica set distribute reads when the system fails. This gives you the flexibility to decide in which situations reads should be prioritized: availability or consistency.
4. **Monitor replication lag**: To spot any delays in data propagation, monitor replication latency between primary and secondary nodes. By guaranteeing timely replication, monitoring this statistic can aid in preventing data discrepancies during failover.
5. **Conduct routine health checks**: Set up a monitoring mechanism to examine your MongoDB replica set's condition on a regular basis. By being proactive, you may identify possible problems before they become serious and take swift action to keep your data consistent.😻
6. **Test failover scenarios**: To learn how your application responds in various circumstances, thoroughly test failover scenarios in a controlled setting. By using this technique, you can find weaknesses in your failover plan and improve data consistency.
By adhering to these best practices, you may improve your Python application's resistance to MongoDB failover events, thereby protecting the consistency and integrity of your data in the face of operational difficulties.
8. Handling connection retries and timeouts in PyMongo for improved failover resilience
It's critical to handle timeouts and connection retries when using PyMongo in your Python program to communicate with MongoDB in order to improve failover resilience. You may improve your application's resilience to interruptions and keep connectivity even in failover situations by setting these options wisely.
In order to incorporate strong connection retry features in PyMongo, think about combining the `auto_reconnect} setting with suitable error management techniques. This functionality minimizes the need for manual intervention during failover occurrences by enabling PyMongo to automatically rejoin when experiencing network problems or server outages.
You can regulate how long a client must wait for a response from MongoDB before deeming an action unsuccessful by adjusting the timeout settings in PyMongo. Changing these settings in accordance with the needs of your application will help keep post-failover communication with the database cluster smooth and avoid long delays.
By using these recommended techniques for managing timeouts and connection retries in PyMongo, you may enable your Python application to gently transition through failover situations, guaranteeing continuous access to MongoDB databases and improving system stability.
9. Advanced techniques for testing failover with large datasets in your Python application
For a thorough assessment, you can use a number of sophisticated ways when working with massive datasets in your Python program to test failover situations. Simulating the failure of primary and secondary MongoDB nodes during intensive read and write operations is one method. You can maximize your application's resilience by doing this and seeing how it responds to various failure scenarios.🥸
Adding packet loss and network latency between your Python program and the MongoDB cluster is an additional method. This will make it easier for you to comprehend how the app handles connectivity problems and resumes operations once the network stabilizes. You can optimize the failover methods in your code and guarantee smooth database node transfers by including these challenges in your testing plan.
During periods of high system stress, you can introduce random problems using tools such as Chaos Monkey. You can identify possible flaws in your failover architecture and take proactive measures to fix them by injecting controlled chaos into your system. Your Python program will become more durable and reliable in production scenarios if you take a proactive approach to testing failover with huge datasets.