1. Introduction
Text overlays are a popular and effective approach to improve visual material. We will look at using Python to add text to photos in this blog article. The ability to apply text overlay to photographs can make communications more engaging and successful whether generating social media visuals, marketing materials, or descriptions for photos. 😎
Text can provoke emotions, provide context, and convey information, which is why adding it to photos is important. You may produce more interesting and educational content that immediately grabs users' attention by integrating text into images. Moreover, text on photos can strengthen branding, draw attention to important details, and increase the shareability of your graphics on many platforms. For developers and creators of digital material, learning how to apply text to images in Python offers up a world of creative possibilities.
2. Setting Up the Environment
Setting up the environment is the first step in using Python to add text to photos. Installing prerequisite libraries is the first step. One such library is Pillow, a well-liked Python image processing library. Using pip, the Python package installer, you may install Pillow by typing the following command into your terminal:
bashpip install Pillow
Setting up your development environment is essential next. Make sure you have a proper environment with the required tools and packages installed to work on your text-on-image project efficiently, regardless of whether you like to use an IDE like PyCharm or Jupyter Notebooks. You'll be able to use Python to easily add text overlays to photographs after your setup is configured.
3. Loading and Displaying Images
When working with images in Python, the Python Imaging Library (PIL) is a powerful tool for loading and manipulating image files. To load an image using PIL, you can use the following code snippet:
```python
from PIL import Image✋
# Open an image file
image = Image.open("image.jpg")
```
You may want to see the image on your screen after it has loaded. Using matplotlib, a well-liked Python charting toolkit with image handling capabilities, is one method to accomplish this. The following code can be used to use matplotlib to display the loaded image:
```python
import matplotlib.pyplot as plt
# Display the image
plt.imshow(image)
plt.axis('off') # Turn off axis numbers
plt.show()
```📖
As an alternative, you can display images in Python using other programs like OpenCV or GUI frameworks like Tkinter. Select the tool that best meets the needs of your project from those available; each offers benefits and applications of its own.
4. Adding Text to Images Programmatically
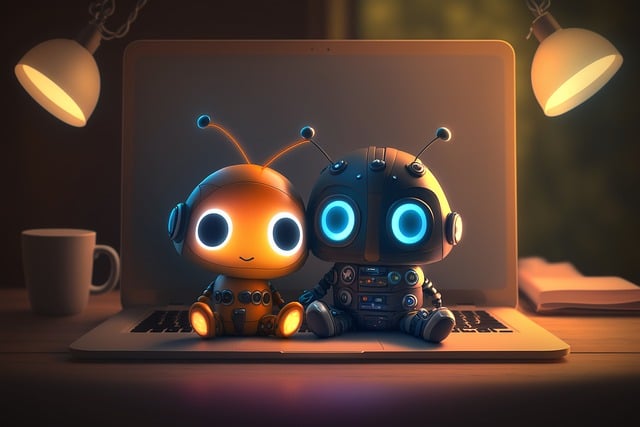
Programmatically adding text to photos can improve their visual appeal and efficiently communicate information. To write text on images in Python, you can use a variety of techniques that offer customization options like changing the font size, color, alignment, and more.
Using libraries like the Python Imaging Library (PIL) or its fork Pillow is one popular technique. Simple routines are available in PIL/Pillow for text drawing on images. Users can fine-tune the text's appearance on the image by adjusting characteristics such font size, color, location, and alignment.
Using packages like OpenCV, which are useful for adding text to photos but are generally used for computer vision tasks, is another well-liked method. OpenCV adds other features including blending modes and anti-aliasing to enable text to blend in seamlessly with the background in addition to text property adjustments.
You may also use libraries such as Matplotlib to add text to photos. Even while Matplotlib is frequently used for creating graphs and charts, it also has features that allow you to add text annotations to photos by adjusting factors like font size, color, alignment, and rotation.
Investigating these various approaches offers flexibility in text addition to images according to particular needs. Python offers flexible methods to meet a variety of demands when it comes to seamlessly embedding textual information on photographs. These needs can include changing the font size for emphasis, choosing colors for contrast or branding consistency, or carefully placing text inside the image composition.
5. Positioning Text on Images
Using Python to create visually appealing and educational visuals requires properly positioning text on images. Coordinates are essential to understanding how to position text on an image. In most image libraries, such as PIL (Python Imaging Library) or Pillow (its successor), the coordinate system usually begins at the image's top-left corner. The standard way to describe coordinates is as (x, y), where x denotes the distance from the image's left edge and y its top edge.
Python approaches that let you determine the right coordinates depending on various parameters like image size, text length, font size, and alignment preferences can be used to position text dynamically on images. One popular method is to figure out the image's center and then modify the text's position accordingly. For exact positioning, you can utilize formulas to determine placements in relation to particular corners or image edges. You may make sure that your text appears where you want it to without having to manually resize each image by cleverly combining these strategies.
You may improve your image processing projects by carefully placing text to offer context and information by learning these positioning strategies in Python. This capacity not only improves beauty but also readability and clarity when it comes to successfully communicating ideas through visual content. By trying out several positioning techniques, you can quickly determine which one is most effective for your particular use case and produce visually attractive photographs with strategically placed text.
6. Saving the Modified Images
After adding text to the photos, it's important to save the updated versions in Python. You can use libraries like PIL (Python Imaging Library) or Pillow, its branch, to store images with additional text. After using these libraries to add the desired text on the image, you can quickly save the edited version to your local drive or share it elsewhere.
Typically, when storing an image with text added in Python, you have to specify both the image quality and file format. Picture formats that are frequently used to save photos are JPEG, PNG, GIF, BMP, and TIFF. File size and image quality are impacted differently by each format. For example, JPEG is a lossy compression technology that shrinks files but may introduce artifacts into the images during the compression process. PNG, on the other hand, is a lossless format appropriate for transparent and sharply edged images.
When deciding on an image format in Python for storing photos with text added, take into account aspects like file size limitations, transparency requirements, and detail sharpness. You can find the ideal ratio of image quality to file size depending on your needs by experimenting with various formats and quality settings.
7. Incorporating Text Effects
You can improve the text's visual attractiveness by giving it shadows, outlines, gradients, or other effects when using Python text effects for images. These effects can either blend the text in perfectly with the image or make it stand out more against different backgrounds.
In Python, you may use libraries like OpenCV or Pillow to give a shadow effect to text. You may build unique shadow effects that enhance readability and compliment the text by adjusting factors such as offset distance and color intensity.
Additionally, outlines can improve how text appears on photographs. You may generate a trendy outline effect that makes the text jump by drawing many copies of the text behind the original text, each with a slight offset and different color.
Another technique to improve the visual appeal of your text is to experiment with gradients. Text may be given depth and dimension by using gradients, which enhances its visual appeal and makes it more intriguing when used on photographs. Programs such as Matplotlib in Python provide features for efficiently applying gradients.
By leveraging these techniques and combining them creatively, you can take your text-on-image projects to the next level, making them more engaging and aesthetically pleasing for your audience.
8. Handling Multiple Images in Batch Processing
Managing several images in batch processing is a typical requirement in the field of image processing. Applying text overlay automatically to a group of photographs can save a lot of time and work. This process can be effectively completed in Python by utilizing packages such as os and Pillow.
To get started, you may write a function that takes in parameters like the text to overlay and the input directory that holds the images. You can also specify the output directory where the modified images should be saved. Iterate over each image in the input directory, apply the necessary text overlay, and save the altered image to the output directory using Pillow's Image and ImageDraw modules.
You may efficiently handle several photos at once and reduce repetitive work by developing batch processing for image text overlay in Python. This method guarantees uniformity across a huge number of photos with text overlays while simultaneously increasing efficiency. Playing around with fonts, sizes, locations, and colors will allow you to further tailor the output to meet your needs.
9. Interactive User Interface for Text Placement
Working with Python image editing tools can be made more enjoyable by including an interactive user interface (UI) for text placement on images. One approach to do this is by using libraries like Tkinter, which is a popular option for Python GUI development because of its ease of use and simplicity, to create a basic graphical user interface. Users can exert greater control over where they want the text to appear on the image by adding a user interface (UI) to your text placement capabilities. 🔧
You can create a window that shows the image and input fields so that users can type text to overlay on top of the image by using Tkinter or other comparable tools. As a result, users may add and modify text elements interactively before applying them to the image, making the experience more dynamic and approachable. Users can further customize their text locations by incorporating elements like alignment options, font selection, size adjustment, and color personalization.
You may make jobs involving the addition of textual information or graphics overlays onto photographs more interesting and productive by providing users with an interactive graphical user interface (GUI) that allows them to experiment with different text positions. An interactive user interface (UI) gives consumers creative freedom over how they present their visual content, while simultaneously streamlining the process. An interactive user interface (UI) for text insertion can improve the overall design possibilities in your Python image editing projects, whether you're adding captions, watermarks, or other comments.
10. Advanced Techniques for Image Text Manipulation
In the realm of advanced image text manipulation techniques in Python, two powerful methods stand out: image segmentation and machine learning models.
For accurate text placement on images, image segmentation is essential. Segmentation helps with more precise text positioning by separating various aspects in a picture, such as objects, backgrounds, and textures, to prevent distortion or overlapping. Applying segmentation techniques such as GrabCut or Mask R-CNN can greatly improve the readability and aesthetic appeal of text overlays on photographs.
Image text manipulation is enhanced when machine learning models are used for intelligent text positioning. Large-scale datasets have been used to train models, which can now automatically identify the best location for text based on layout, aesthetics, and picture content. Natural language processing and object detection are two techniques that can be used to evaluate image-text relationships and create visually appealing compositions that smoothly integrate text and images.
You can improve the quality of your picture text transformations and produce visually appealing images that effectively convey your message by using these sophisticated approaches in your Python applications.
11. Adding Watermarks to Images
It's normal practice to add watermarks to photos in order to assert copyright and protect your work. Using packages like PIL (Pillow), you may create transparent watermarks with personalized text with ease in Python. You may make sure that people who view your photographs know that they are yours and contain copyright information by including a watermark.
In Python, you must first load the image using PIL before you can add a watermark. After that, you can make a new image with the desired watermark text and alter its transparency level, color, and font size. To generate the final watermarked image, you can then overlay this watermark image onto the original image.
By including your watermark with copyright information, you can protect your ownership of the image and stop illegal use. This makes it very evident that the image is yours, protecting your intellectual property. You can create a barrier against unauthorized use and reproduction of your photos by affixing copyright information to them.
As I mentioned earlier, one of the most important steps in protecting your photos online is to apply watermarks with personalized text and copyright information. Watermarking is a simple and efficient operation when using Python modules such as PIL (Pillow). You may protect ownership rights and deter unlawful use while keeping a professional appearance by adding these components to your photos.
12. Conclusion
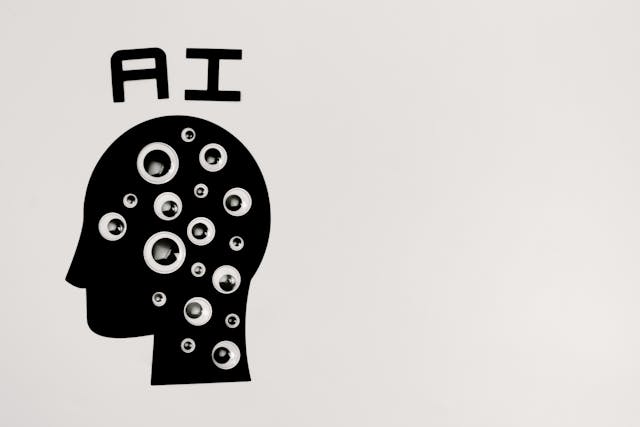
After putting everything above into context, we can say that adding text to photos with Python is a flexible technique to improve visual content. This procedure creates innovative opportunities for quickly and programmatically overlaying photographs with information, branding, or context. We can dynamically change images and text to create unique visuals for a variety of applications, like social media posts, advertising materials, or presentations, by integrating libraries like OpenCV and PIL with pertinent Python code snippets.
Understanding the fundamentals of managing images in Python, using libraries like OpenCV and PIL for image processing tasks, and smoothly incorporating text overlay functionality into images are the main lessons to be learned from this tutorial. By using this technique, users can personalize the design components to suit their tastes and automate the process of adding text to photographs at scale. Examining more complex options like as blending modes, positioning strategies, and font styling might improve the output's visual impact even further.
If you're keen to learn more about this topic, you might like to try with some other features like dynamic text placement using picture recognition algorithms or creating interactive visual content with API integrations. Using a hands-on approach and experimentation to discover new methods to successfully use text on images not only strengthens learning but also ignites creativity. Keep in mind that there are countless options available when using Python's extensive library and toolkit for image processing jobs.