1. Introduction
Welcome to Java's Coffee Talk, a series where, while sipping coffee, we explore the world of Java programming. We will solve the mystery behind five typical runtime issues that Java developers frequently come across in this session. If a Java programmer wants to develop reliable and error-free code, they must understand these errors. So grab a drink of your choice, settle in, and let's work together to decipher these runtime issues.
2. Runtime Error: NullPointerException
Runtime Error: NullPointerException is one of the most common errors in Java programming. It occurs when a program tries to access or modify a reference variable whose value is
3. Runtime Error: ArrayIndexOutOfBoundsException
When attempting to access an index outside of an array's bounds, Java throws the Runtime Error: ArrayIndexOutOfBoundsException. This error usually occurs when you try to access an element of an array at a point that is either larger than or equal to the array's length, or less than 0.
Consider the following sample code snippet that demonstrates a scenario where ArrayIndexOutOfBoundsException might occur:
```java
public class ArrayIndexErrorExample {
public static void main(String[] args) {
int[] numbers = new int[5];
// Accessing an element outside the bounds of the array
int invalidIndex = numbers[5];
}
}
```🖊
To avoid ArrayIndexOutOfBoundsException, always ensure that the index used to access elements in an array is within the valid range. Here are some suggestions for preventing and managing this error:
1. Check the bounds: Before accessing any element in an array, verify that the index lies within the bounds of the array (from 0 to length-1).
2. Use loops carefully: When iterating over arrays using loops like for or while, be cautious with loop conditions to avoid going beyond the last index.
3. Deal with special cases: If failures are possible, use try-catch blocks to catch ArrayIndexOutOfBoundsException and treat them graciously by giving suitable error messages or fallback methods.
4. Validate user input: When dealing with user-provided indices for array access, validate and sanitize inputs to make sure they are valid before using them directly.
Developers can efficiently prevent and handle ArrayIndexOutOfBoundsException in their Java projects, resulting in more robust and dependable codebases, by adhering to certain best practices and being aware of array boundaries.
4. Runtime Error: ClassCastException
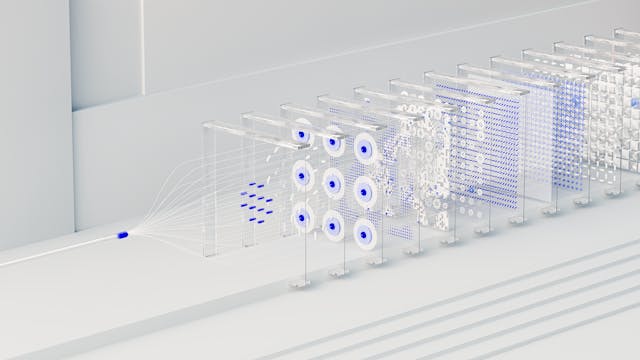
Dealing with a ClassCastException during runtime can be a frequent problem for Java programmers. When an object is attempted to be cast to a subclass of which it is not an instance, an error occurs. Since objects in Java can only be cast to types that exist in their class hierarchy, ClassCastException indicates that there is a problem with the type casting procedure.
To illustrate, consider the following code snippet:
```java
List<String> stringList = new ArrayList<>();
stringList.add("Java");
// Attempting to cast a String list to an Integer list results in ClassCastException
List<Integer> integerList = (List<Integer>) (List<?>) stringList;
```
Because of the incompatible types in this example, attempting to cast a {List
Here's how you can employ generics for safer type conversions:🥰
```java
public <T> List<T> convert(List<?> list) {
List<T> result = new ArrayList<>();🧭
for (Object obj : list) {
if (obj instanceof T) {
result.add((T) obj);
}
}
return result;
}
```
When working with type conversions in Java, developers can reduce the likelihood of ClassCastException problems and guarantee more seamless runtime operations by utilizing generics and verifying type compatibility with {instanceof}.
5. Runtime Error: ConcurrentModificationException
Getting a ConcurrentModificationException can be a regular problem while working with Java programming. When you attempt to update a collection while concurrently iterating over it, you get this error. Java developers must comprehend how this problem occurs and be aware of the best ways to avoid or fix it.
Adding or removing elements from a collection while using an iterator on the same collection, altering a collection directly during an iteration with an enhanced for loop, or utilizing multiple threads to modify the same collection without proper synchronization are examples of instances that frequently result in ConcurrentModificationException.✍️
Consider utilizing iterators correctly and refraining from making changes to collections during iteration to prevent ConcurrentModificationExceptions in your Java project. As an alternative, you can utilize concurrent collections that are safe to handle concurrent updates, such `ConcurrentHashMap` or `CopyOnWriteArrayList`, which are supplied by Java's `java.util.concurrent} package.
You can successfully prevent or resolve ConcurrentModificationExceptions in your Java projects, ensuring more reliable and smooth code execution. Some recommended practices to consider are using concurrent collections when necessary or adhering to appropriate synchronization.
6. Runtime Error: IllegalArgumentException
A Java runtime error known as a `IllegalArgumentException` is raised when an argument passed to a method is not within a specified range or is not of the anticipated type. This exception usually means that something is wrong with the arguments that are being supplied to the method.
Instances where `IllegalArgumentException` can occur include passing
7. Conclusion
We've covered five common runtime problems in Java thus far: NullPointerException, ArrayIndexOutOfBoundsException, ClassCastException, ConcurrentModificationException, and OutOfMemoryError. Let me summarize what I've written so far. For Java programming to be effective, it is essential to comprehend these mistakes. Developers are able to design more reliable and error-free code when they are aware of potential problems and how to solve them.
Maintaining awareness and being ready are crucial for properly addressing these runtime problems. Java programs that use defensive code, validate inputs, handle errors gracefully, and keep an eye on memory utilization are much less likely to have these problems. Better software stability and more efficient development processes can be achieved by adopting best practices and exercising caution when developing.
We want our readers to offer any more advice or insights they may have, as well as their experiences with Java runtime problems. Community feedback enhances our collective understanding and fosters our own development as developers. Through participating in conversations on typical code problems, such as runtime faults, we may provide a welcoming environment for learning and developing Java abilities. We appreciate you coming along as we explore the subtleties of Java's coffee talk with these five runtime faults that have been decoded!