1. **Introduction**
In Hadoop Development with MapReduce, bulk deletion of column values refers to the process of removing multiple entries within a specific column across large datasets efficiently. This operation is crucial for maintaining data integrity and optimizing storage space within the Hadoop ecosystem. Efficiently managing large datasets in Hadoop is essential due to the massive volume of information processed daily. The ability to swiftly delete unnecessary or outdated entries helps improve query performance, reduce storage costs, and streamline data analysis processes. Proper management ensures that the system runs smoothly and maximizes its potential for processing big data effectively.
2. **Overview of MapReduce**
Google developed the MapReduce programming style and framework to handle massive volumes of data in parallel over a dispersed cluster. In the context of Hadoop, MapReduce is essential for efficiently allocating processing work among cluster nodes in order to manage large datasets. The Map phase, which divides data into smaller chunks for parallel processing, and the Reduce phase, which aggregates the output from the Map phase, make up the framework's two primary stages.
Reducing the complexity of processing and analyzing large datasets is one of the main advantages of utilizing MapReduce in Hadoop development. MapReduce helps developers to take use of distributed computing to achieve quicker processing speeds and improved resource efficiency by breaking down large, data-intensive activities into smaller, more manageable jobs that can be performed in parallel. This method is perfect for big data applications since it increases scalability and performance simultaneously.
By utilizing distributed computing principles, MapReduce essentially offers a scalable and fault-tolerant framework for managing large-scale data processing jobs. Because of its ease of use and efficiency, it is becoming a vital part of Hadoop ecosystems for creating reliable and effective data processing pipelines.πΆβπ«οΈ
3. **Bulk Deletion in MapReduce**
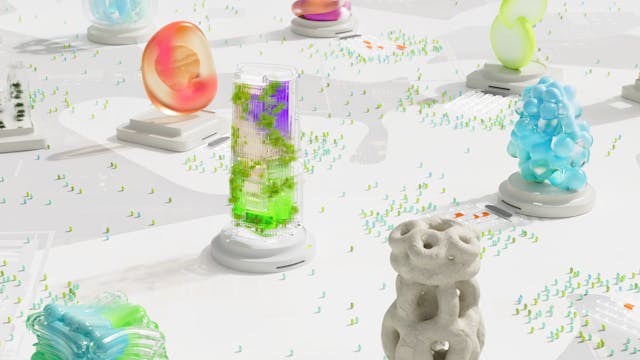
Maintaining big data applications in Hadoop Development using MapReduce requires bulk elimination of column values. The ongoing evolution of datasets and the efficient cleaning or updating of particular columns necessitate mass deletion. Organizations can guarantee data accuracy, consistency, and overall system performance by mass deleting old or incorrect values.
But carrying out large-scale bulk deletions presents a number of difficulties. The sheer amount of data that must be processed and changed at the same time presents a major obstacle. The difficulty and time needed for deletion operations increase with dataset size. Another big issue is ensuring data integrity while deleting information in bulk. It takes careful handling to ensure consistency between various dataset segments when removing particular column values in order to avoid data loss or corruption.
4. **Implementing Bulk Deletion using MapReduce**
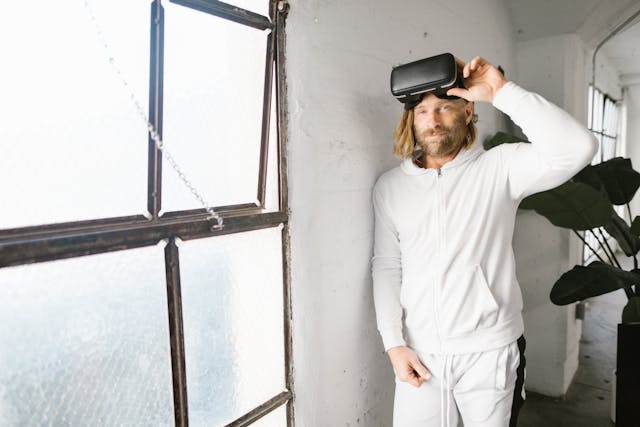
Using MapReduce to implement mass deletion of column values in Hadoop development entails segmenting the procedure into manageable phases. Determine which columns in the dataset should be removed first. Create a MapReduce job after that, which will loop through the data and remove any columns that aren't needed. Writing unique mappers and reducers to manage the filtering logic can help achieve this.
Establishing your input and output key-value pairs is the first step in utilizing MapReduce to accomplish mass deletion. Usually, the row ID would serve as the input key, and all of the columns connected to that row would be represented by the value. Parse each input line in your mapper function, then use established criteria to filter out the columns you don't want. The filtered columns can then be combined again by the reducer into a structured output.
Code snippets are crucial in understanding this process better. Below is an example illustrating how you can implement bulk deletion of column values using MapReduce:
```java
public class ColumnDeletionMapper extends Mapper<Object, Text, Text, Text> {
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
// Parse input line
String[] columns = value.toString().split(",");
// Perform column deletion based on condition
StringBuilder outputColumns = new StringBuilder();
for (int i = 0; i < columns.length; i++) {π
if (/* Add condition for column deletion */) {
continue;
}
outputColumns.append(columns[i]).append(",");
}
π’
context.write(new Text(/*row ID*/), new Text(outputColumns.toString()));
}
}
public class ColumnDeletionReducer extends Reducer<Text, Text, Text, Text> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
for (Text value : values) {
context.write(key,value);
}
}
}π₯°
```
`ColumnDeletionMapper` in this code snippet analyzes each row of data and eliminates unneeded column values in accordance with a predetermined requirement. For every row, the `ColumnDeletionReducer` subsequently combines the filtered columns once more.
Implementing mass deletion of column values in Hadoop development with MapReduce gets easier and more accessible by following these steps and using code samples such as the one above. Large datasets can be handled effectively with this method, which also makes sure that only pertinent data is kept for later processing or analysis.
5. **Handling Large Datasets Efficiently**
Using MapReduce to delete column values in bulk in Hadoop requires handling huge datasets with efficiency. The key to optimizing deletion operations for large datasets is to use methods that can manage the amount of data while maintaining efficiency. Using partitioning and indexing techniques to target particular data subsets for deletion is one way to lessen the system's overall workload.
Utilizing incremental processing techniques, which divide the deletion task into smaller, more manageable pieces that may be handled separately, is another tactic. The deletion process can be made more parallelizable by partitioning the dataset into batches or partitions. This allows for faster execution times and less resource strain.
Effective data compression techniques can assist reduce the amount of storage needed for the deletion process, which will cut down on processing time and disk space use. Through the optimization of data storage and retrieval strategies within Hadoop, developers can expedite the removal of column values from huge datasets while preserving scalability and speed.
6. **Testing and Debugging**
In the development of MapReduce jobs, testing and debugging are essential stages, especially when working on tasks such as bulk deletion of column values. In order to guarantee process correctness and efficiency, developers have a number of options.
Prior to executing the job on larger data sets, a good approach is to run the test cases using smaller input datasets. This lowers the possibility of problems happening on a large scale by enabling developers to find and fix faults in a controlled setting. Validating the logic of mapper and reducer functions can be facilitated by using tools such as MRUnit for unit testing.
A crucial tool for debugging is logging. By integrating extensive logging capabilities into the MapReduce task, developers may monitor data flow and spot possible mistakes or bottlenecks. Through log analysis, engineers may promptly identify problems and enhance overall performance.π
Debugging can also be facilitated by implementing checkpoints at critical points throughout the job execution. Developers can confirm the accuracy of operations up to that point and identify trouble spots if mistakes arise later in the process by strategically storing intermediate outputs.
Following coding standards is essential when it comes to best practices for guaranteeing accuracy and effectiveness during implementation. Coding in a clean, modular manner improves readability and makes debugging easier by focusing problems on individual components.
Early in the development cycle, possible problems or inefficiencies might be found by conducting in-depth code reviews with peers. Within the development team, collaborative feedback fosters continual progress and guarantees adherence to best practices.
Finally, performance tweaking is essential to MapReduce job optimization. Through optimization of variables like input splits, combiner functions, and memory settings, developers can achieve substantial processing time reductions and increased efficiency.
To summarize my previous writing, when creating MapReduce jobs for activities like bulk column value elimination, testing and debugging are essential stages. Developers may achieve high-quality MapReduce solutions and expedite development processes by adopting best practices for accuracy and efficiency, optimizing performance, and putting strong testing procedures into practice. π
7. **Performance Tuning**
When handling bulk deletion of column values in Hadoop using MapReduce, optimizing performance is crucial. Here are some tips for improving performance:
1. **Partitioning**: Utilize data partitioning techniques to distribute the workload evenly across nodes. This helps in reducing the processing time by enabling parallel processing.
2. **Combiners**: Implement combiners to perform local aggregation before sending data to reducers. This reduces the amount of data shuffled across the network and minimizes the load on reducers.
3. **Map-side Joins**: If appropriate, think about combining datasets early in the map phase as opposed to later in the reduction phase by employing map-side joins. Because less data is sent between mappers and reducers, performance can be greatly increased.
4. **Optimized Input Formats**: Choose optimized input formats such as SequenceFile or ORC to enhance read efficiency and reduce disk I/O operations.π±
5. **Data Compression**: Compress intermediate data outputs to reduce disk space usage and speed up data transfer between mappers and reducers.
6. **Tuning Memory Configuration**: To optimize resource use and avoid memory-related bottlenecks, modify memory parameters such as heap size, buffer sizes, and memory allocation settings.
You can increase the effectiveness of bulk deletion operations in Hadoop development using MapReduce by utilizing these performance tuning strategies, which will result in quicker execution times and better system performance overall.
8. **Real-world Use Cases**
The mass deletion functionality in Hadoop development projects has several real-world applications in a variety of industries. For example, effective mass deletion can assist assure compliance with data privacy rules like HIPAA by swiftly eliminating obsolete or superfluous material in the healthcare sector, where large amounts of sensitive patient data are held.
In order to manage enormous datasets of customer call records or network logs, telecommunications organizations also make use of bulk deletion capabilities. This helps them to maximize data processing performance and simplify storage resources. In situations with this kind of heavy traffic, this is essential to keeping the infrastructure responsive and lean.
E-commerce sites use Hadoop's mass deletion feature to rapidly purge out-of-stock items and old listings from their product databases. They increase the relevancy of search results, improve user experience, and efficiently handle inventory data by doing this.
Bulk deletion features help financial companies stay compliant with stringent regulations like GDPR and PCI DSS while keeping accurate and current information. In addition to ensuring industry compliance, automating the deletion of outdated financial transactions or client information reduces the possibility of data breaches.
Organizations in a variety of industries can efficiently improve data management procedures, guarantee regulatory compliance, and maximize operational efficiency thanks to Hadoop development's mass deletion capabilities.
9. **Security Considerations**
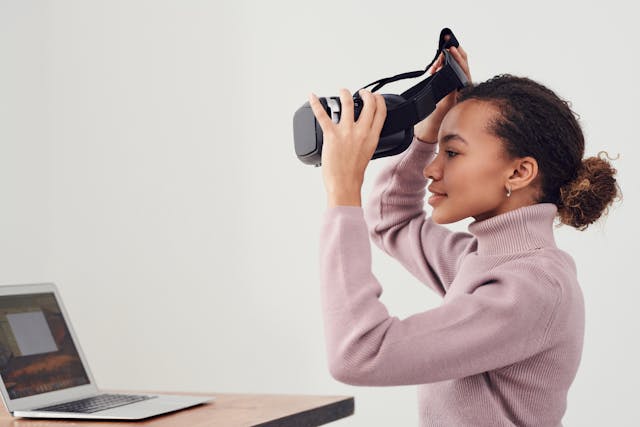
Security issues are critical when using MapReduce to delete column values in bulk in Hadoop. In a distributed system such as Hadoop, deleting data necessitates meticulous planning in order to protect data integrity and stop unwanted access. Making sure that only authorized users have the right permissions to start bulk deletion actions is an important step.
Implementing strong authentication and authorization systems is crucial to addressing security risks associated with mass deletion in Hadoop. Only trusted persons or groups should be granted access privileges, and user access control should be carefully enforced. Additionally, encryption methods can be used to safeguard sensitive data while it is being deleted and to ensure safe communication between nodes.πΉ
It is necessary to implement auditing and logging procedures in order to keep track of and oversee any bulk deletion operations. This makes it easier to quickly see any questionable activity or illegal access attempts. Frequent security audits and assessments can also assist in identifying possible weak points and guarantee that the system is protected from changing threats.
Through the integration of security measures into the bulk deletion procedure in MapReduce Hadoop development, entities may ensure the protection of their data assets and minimize the potential consequences of unapproved data removal or manipulation.
10. **Comparison with other Approaches**
When comparing huge data processing bulk deletion techniques, MapReduce sticks out as a conventional yet successful strategy. But as big data frameworks have developed and technology has advanced, new strategies have appeared that provide quicker and more effective means of handling mass deletion jobs.
Apache Spark is an alternative to MapReduce for mass deletion. Large datasets can be processed more quickly with Spark's in-memory computing capabilities than with MapReduce's disk-based processing. Spark is a popular option for businesses trying to optimize their data processing workflows because it produces faster deletion operations.
Utilizing Apache Flink for mass deletion jobs is another strategy that is gaining popularity. Flink is a good choice for real-time data processing needs because of its low latency and high throughput processing. When managing constant additions and deletions inside a dataset, its effective streaming data handling capabilities can be useful.
Incremental data processing capabilities are provided by technologies such as Apache Hudi, which can be utilized for effective bulk deletion procedures. Hudi facilitates targeted deletions by allowing the removal of particular records or partitions from datasets without requiring the full dataset to be processed again, hence saving time and resources.
While MapReduce is still a solid choice for bulk deletion in Hadoop development, investigating other strategies like Spark, Flink, and Hudi can provide improved efficiency and performance depending on certain use cases and requirements in pipelines for big data processing.
11. **Future Trends and Challenges**
**Future Trends and Challenges**
Future developments in MapReduce bulk deletion procedures on Hadoop platforms are anticipated to concentrate on improving performance, scalability, and efficiency as the big data space continues to develop. Using machine learning techniques to optimize deletion activities based on past data trends is one possible advancement. Developers may be able to automate and optimize the deletion process for increased speed and accuracy by utilizing predictive analytics.
The creation of specialized tools or frameworks intended especially for mass deletion operations in Hadoop systems is another trend that can take off. To make the deletion process easier for developers, these solutions could come with pre-built features including sophisticated filtering options, job scheduling mechanisms, and real-time monitoring capabilities.
But these developments also bring new difficulties. Ensuring data consistency and integrity during bulk removals is a major concern. While erasing massive amounts of data from distributed systems, developers must have strong error handling methods in place to guard against data corruption or loss.
Optimizing deletion processes to reduce resource consumption and execution time will continue to be a major challenge as datasets get bigger and more complex. To achieve high-performance bulk deletion operations on Hadoop platforms, creative solutions to efficiently parallelize deletion activities over several nodes without sacrificing system stability will be crucial.
12. **Conclusion**
To sum up, using MapReduce to execute bulk deletion of column values in Hadoop Development provides a scalable and effective way to manage enormous volumes of data. This procedure expedites data modification processes, increases processing speed, and enhances data quality. Organizations can improve their big data operations and data management procedures by utilizing MapReduce's capabilities. The importance of this method should be emphasized since it has the ability to completely change how businesses manage and analyze data in their Hadoop clusters.βοΈ