1. Introduction
In computer science, it is essential to comprehend the differences between Divide and Conquer and Dynamic Programming in order to maximize problem-solving techniques. A issue can be divided into smaller, more manageable subproblems using the divide and conquer strategy. These subproblems can then be solved recursively and combined with one another to solve the main problem effectively. The goal of dynamic programming, on the other hand, is to divide an issue into overlapping subproblems, solve each subproblem just once, and store the solution to prevent repeating calculations. Both methods are useful for designing algorithms and have their advantages. Understanding the differences between them enables developers to select the best strategy for a particular issue, maximizing algorithmic solutions' efficacy and efficiency.
2. Definition
**Definition**✍️
Divide and conquer is a technique for tackling challenges in which a difficult problem is divided into smaller, easier-to-manage subproblems. The original problem is solved by combining the solutions to several independently solved subproblems. Usually, the procedure consists of three stages: break the problem up into smaller components, solve these components individually, and then combine the answers to obtain the whole solution.
Conversely, dynamic programming is a technique for resolving complicated issues by decomposing them into more manageable subproblems. However, dynamic programming saves the answers to subproblems in a table to avoid recalculating them, in contrast to divide and conquer, which solves each subproblem separately. As a result, computation can be done more quickly by avoiding unnecessary calculations.
**Similarities and Differences**
Both strategies divide issues into smaller components in order to simplify the solution process, but they handle overlapping subproblems differently. Since Divide and Conquer does not save interim results, if a subproblem occurs more than once, there may be computational redundancy. On the other hand, Dynamic Programming streamlines this procedure by keeping track of subproblem solutions in a table, guaranteeing that each subproblem is resolved just once.
Another key difference lies in their applications: Divide and Conquer is often used when subproblems do not overlap or share resources, making it suitable for tasks like sorting algorithms (e.g., merge sort). Dynamic Programming shines when there is optimal substructure among subproblems - meaning an optimal solution can be constructed from optimal solutions of its subproblems - as seen in problems like shortest path calculations or sequence alignment.
Essentially, both approaches use decomposition to simplify complicated issues, but they differ in how they handle overlapping subproblems and store intermediate states, making them more effective and applicable in various contexts.
3. Algorithm Design
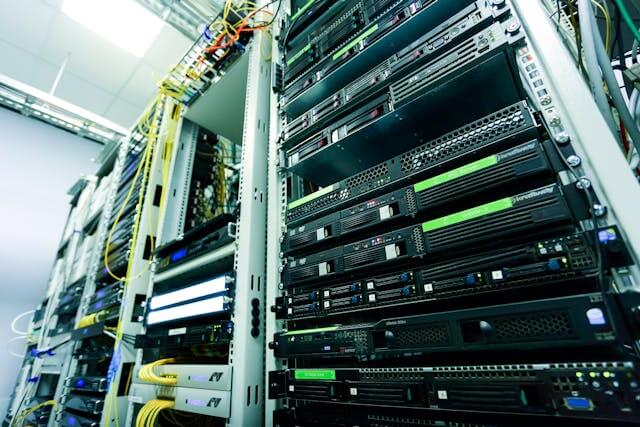
Divide and Conquer techniques in algorithm design entail segmenting an issue into smaller, more manageable pieces, addressing each one separately, and then merging the solutions to address the original problem. Three phases are usually included in this approach: breaking the problem down into smaller problems, solving these smaller problems iteratively, and merging the results.
However, in order to save repeating computations, Dynamic Programming concentrates on storing intermediate subproblem results. It works by starting with smaller subproblems and working its way up to larger ones. This method is especially helpful when there are optimal substructure qualities inside the problem or when subproblems overlap.
For instance, in the traditional Divide and Conquer sorting algorithm known as Merge Sort, the array is divided in half, each half is sorted independently (conquered), and the sorted halves are then combined. On the other hand, when utilizing dynamic programming to calculate the Fibonacci sequence, we store the Fibonacci numbers that have already been determined in an array to save time on subsequent calculations.
4. Application Areas
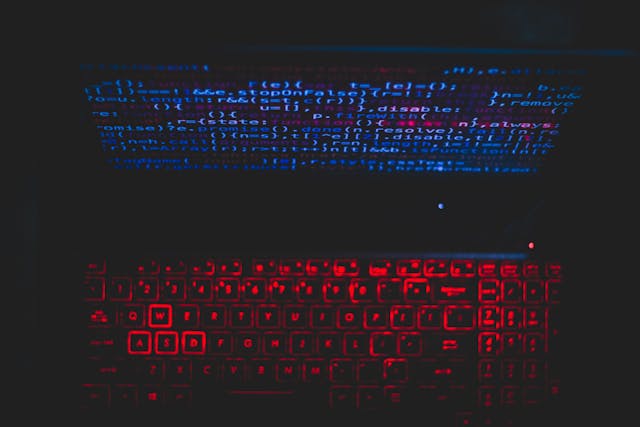
Divide and Conquer is frequently employed in a variety of real-world applications, including parallel computing jobs that may be divided into smaller, independent subtasks and sorting algorithms (such binary search and merge sort, quicksort). By dividing complex issues into smaller, more manageable components, solving each component separately, and then integrating the solutions to get the answer, this method frequently shows to be effective when dealing with large-scale difficulties.
On the other hand, Dynamic Programming is more suitable than Divide and Conquer in scenarios where subproblems overlap or share optimal substructures. It is commonly applied in tasks like sequence alignment (e.g., DNA sequence comparison), shortest path problems (e.g., Dijkstra's algorithm for graphs), and optimizations related to cutting stocks, scheduling, or resource allocations where the optimal solution of larger problems depends on the optimal solutions to smaller instances.
In terms of the two approaches' suitability for use in various problem domains, Divide and Conquer works best in situations where the issue can be split up into discrete subproblems with distinct solutions. On the other hand, where there are overlapping subproblems that can maximize computing efficiency by reusing previously computed solutions, dynamic programming excels. For improved efficiency and scalability, it is essential to comprehend these differences in order to choose the best algorithmic solution based on the specific aspects of the problem.
5. Efficiency Analysis
It's important to take into account how each method handles subproblems when comparing the temporal complexity of Dynamic Programming techniques with Divide and Conquer algorithms. To find the ultimate solution, Divide and Conquer usually divides the problem into independent subproblems that are solved recursively and then combined. O(n log n) or O(n^2) time complexity is frequently the result of this process, depending on the algorithm and issue type.
Dynamic programming, on the other hand, divides the problem into overlapping subproblems, saves the solutions to prevent repeating calculations, and works its way up to solving more complex subproblems. In many circumstances, this bottom-up method can result in a time complexity of O(n^2) or better. Both strategies seek to tackle problems by dissecting them into smaller components, but depending on the particulars of the issue at hand, their efficaciousness may differ dramatically.
The decision between dynamic programming and divide and conquer frequently comes down to the specifics of the issue at hand. Divide and Conquer works well in situations when the subproblems are genuinely independent of one another, like in Merge Sort or Quick Sort sorting algorithms. In many situations, breaking the problem up into manageable chunks might result in noticeable performance increases over alternative approaches.
However, dynamic programming excels in solving issues with overlapping subproblems that stand to gain from intermediate result storage or memoization. For example, the Floyd-Warshall algorithm or Fibonacci sequence computations show how the caching capability of Dynamic Programming can significantly increase efficiency when compared to Divide and Conquer tactics.
The distinctions between these approaches may become hazy in some situations, necessitating careful consideration in order to choose the best course of action. It is important to take into account variables such as optimal substructure, overlapping subproblems, memory restrictions, and trade-offs between space and time difficulties in order to determine when divide-and-conquer or dynamic programming should be used.
Dynamic programming is frequently used in real-world situations for optimization jobs when identifying the best answer necessitates effectively examining a range of options. Issues like matrix chain multiplication and knapsack optimization serve as examples of how dynamic programming's capacity to retain interim results produces better outcomes than simpler divide-and-conquer strategies.🗞
On the other hand, because of its inherent parallelizability across disjoint subproblems, it may perform better than its dynamic programming counterparts when tackling divide-and-conquer-friendly problems like quicksort, where partitioned segments are entirely independent during recursion processes, or parallelizable tasks that benefit from splitting work among multiple processors without shared global data dependencies.
Consequently, choosing between Divide and Conquer and Dynamic Programming requires a detailed comprehension of the structure of the problem and the interdependencies between the solutions of the subproblems; this knowledge enables the selection of an algorithmic paradigm that is optimized for maximizing computational efficiency given the constraints and goals.
6. Space Complexity Comparison
Divide & Conquer usually demands more memory than Dynamic Programming in terms of space complexity. This is a result of Divide & Conquer's memory-based storage of numerous subproblems until they are resolved and combined. Depending on the problem structure, Divide & Conquer algorithms frequently have space complexity of O(n) or O(log n).
On the other hand, by storing and reusing subproblem solutions, dynamic programming maximizes the use of available space. For many situations, this results in a lower space complexity—typically O(n) or O(n^2)—than Divide & Conquer, making it more memory-efficient.
Take the computation of the Fibonacci sequence using both methods, for instance. Whereas each recursive call in Divide & Conquer results in more stack frames and a higher space complexity, in Dynamic Programming, the previous values are stored in only two variables, requiring a significantly smaller amount of space.
7. Relationship Between the Two Techniques
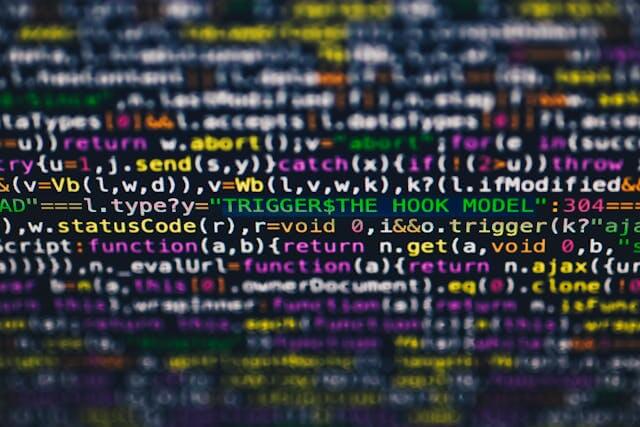
To increase efficiency, Divide and Conquer techniques can occasionally be used into Dynamic Programming solutions. With the help of this fusion, an issue may be divided into smaller subproblems utilizing Divide and Conquer strategies, and the solutions can then be effectively stored and reused by employing Dynamic Programming. In doing so, it makes better use of the advantages of both approaches to address complicated problems.
For the purpose of resolving complex issues, hybrid systems that incorporate components of both Divide and Conquer and Dynamic Programming techniques have grown in popularity. These strategies could include breaking the problem down into smaller, more manageable components using the Divide and Conquer principles, using Dynamic Programming to solve these smaller problems quickly, and then merging the solutions to arrive at the ultimate answer. The outcome of this integration is frequently more optimized algorithms that achieve a balance between space efficiency and temporal complexity.
Take a look at an example similar to the computation of the Fibonacci sequence to see how they complement each other. Even though a simple recursive method divides the problem into smaller subproblems in accordance with the Divide and Conquer principle, the overlapping subproblems cause superfluous recalculations in the method. We can do away with this inefficiency and still take advantage of the basic divide-and-conquer character of the problem by implementing memoization or bottom-up dynamic programming approaches. This hybrid approach demonstrates how these two approaches can work in concert to produce the best results.
8. Performance Trade-offs
It's important to take into account situations in which Divide and Conquer performs better than Dynamic Programming when comparing the two approaches in terms of performance. Divide and Conquer frequently performs well on tasks that lend themselves to easy parallelization, enabling quicker processing times. However, when there are overlapping subproblems that may be saved and utilized again to increase efficiency, dynamic programming really shines.
Because Divide and Conquer breaks the problem up into smaller subproblems, it requires less memory to retain intermediate findings, which results in a lower memory footprint. However, because dynamic programming saves answers to subproblems in a table or array for subsequent use, it may need more memory.
It is important to consider the trade-offs between these approaches in light of the particulars of the problem at hand. Dynamic programming can significantly increase speed for issues where there is a large overlap between the ideal substructure and subproblems by avoiding duplicate computations. On the other hand, while Divide and Conquer can process information in parallel, it might perform better if a problem can be effectively split up into separate subproblems with unique solutions. One can decide which strategy best fits the problem's criteria for speed or memory economy by being aware of these subtleties.
9. Implementation Challenges
Ignoring the base case in recursive functions is a typical mistake made when implementing Divide and Conquer solutions. Inadequate base case definitions might result in inaccurate or infinite recursive outcomes. Maintaining efficiency and clarity while decomposing the problem into smaller subproblems is another challenge, since the validity of the algorithm as a whole depends on how well these subproblems are managed.
One of the main challenges in dynamic programming is figuring out what the problem's ideal substructure is. Though it can be difficult, figuring out how tiny subproblems relate to one another and help solve the bigger problem is crucial. Efficiently managing overlapping subproblems can be challenging because the algorithm's performance can be greatly impacted by recalculating the same subproblem several times.
Implementations of Divide and Conquer must carefully define base cases and make sure they are handled correctly in recursive functions in order to address these difficulties. Implementation complexity can be reduced by logically and effectively breaking down larger problems into smaller subproblems. Effective identification and correction of implementation faults can also be facilitated by doing comprehensive testing under various scenarios and input sizes.
Clarity on how subproblems interact inside the algorithm can be obtained in a Dynamic Programming setting by obtaining a thorough grasp of the problem's optimal substructure through analysis or visualization. By getting rid of pointless computations, applying strategies like memoization or tabulation to save and reuse calculated results of overlapping subproblems can greatly improve performance. Effective Dynamic Programming implementations require regular reviews and optimizations of the cached results storage and retrieval techniques.
Divide and Conquer implementation problems require painstaking attention to detail when building recursive structures and efficiently managing subproblem divisions. Overcoming challenges in Dynamic Programming necessitates a thorough understanding of the best substructures within problems along with tactical methods like memoization or tabulation to maximize efficiency while effectively managing overlapping subproblems. Through proactive resolution of these common issues and the adoption of best practices specific to each technique, developers can greatly improve the efficacy of the algorithms they apply.
10. Decision-Making Factors
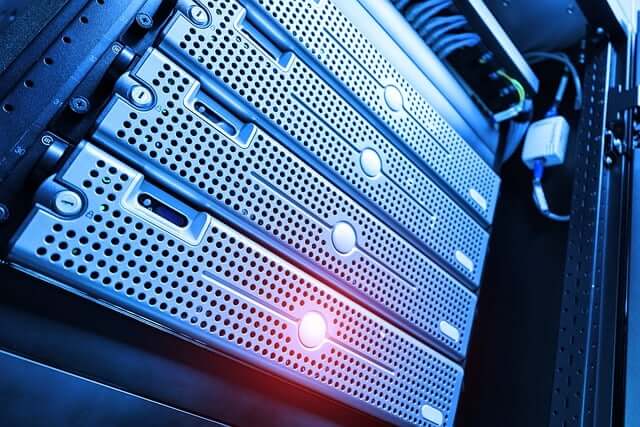
The decision between Divide and Conquer and Dynamic Programming is influenced by a number of important considerations. The nature of the issue at hand is one important factor. Dynamic programming is better suited for issues with overlapping subproblems that display optimal substructure, but divide and conquer is frequently chosen when the problem can be simply split into subproblems that are independent of one another.
The problem's temporal complexity requirements influence the technique selection as well. Due to its ability to divide a problem into smaller, independent subproblems, Divide and Conquer usually works effectively for problems with exponential or logarithmic temporal complexity. Dynamic programming, on the other hand, works well to reduce temporal complexity by preventing unnecessary calculations and saving solutions to overlapping subproblems.
One last thing to think about is memory use. Because dynamic programming stores intermediate results in a table or matrix, it typically uses more memory than divide and conquer. Divide and Conquer might be a preferable choice if memory is an issue because it only records the answers to each subproblem until they are merged at the conclusion.
The choice between these two methods depends critically on whether the problem has an optimal substructure. This property—that an optimal solution can be effectively generated from optimal solutions of its subproblems—is the foundation of dynamic programming. In the event that a problem lacks this quality, Divide and Conquer may be a better solution.
A number of considerations should be taken into account while choosing between Divide and Conquer and Dynamic Programming, including the issue structure, time complexity requirements, memory use limitations, and the existence of an ideal substructure. Through a thorough evaluation of these factors, you may select the approach that will best address your particular problem.
11. Case Studies
Case studies are incredibly useful resources for both practical application and better understanding in the field of algorithm design. We may extract insights that not only confirm the effectiveness of Divide & Conquer and Dynamic Programming approaches, but also offer important takeaways for future algorithmic initiatives by examining particular cases where these strategies have been applied successfully.
We can see the effectiveness of Divide & Conquer tactics in action by examining case studies employing these strategies. This method is demonstrated, for example, by the merge sort algorithm, which sorts huge datasets quickly. Merge sort algorithms are elegant and effective when one understands how they split down a large sorting assignment into smaller subproblems, solve them separately, and then merge these answers back together.
However, studying case studies that make use of dynamic programming illuminates its special advantages. Take into consideration the well-known example of calculating the Fibonacci sequence with dynamic programming. Dynamic programming greatly reduces time complexity by saving intermediate outcomes to prevent duplicate computations. Analyzing such scenarios offers lucid examples of how this methodology might efficiently address issues with overlapping subproblems.
These case studies provide priceless insights on algorithm design. Recognizing patterns that lend themselves to Divide & Conquer or Dynamic Programming techniques becomes essential when dealing with complex situations. Through the identification of commonalities between novel difficulties and previous achievements, designers can make well-informed judgments regarding the methodology to utilize, thus optimizing workflow and augmenting productivity.
Examining Divide & Conquer and Dynamic Programming in real-world contexts through case studies provides not only theoretical understanding but also useful insights that can influence algorithm development in the future. We arm ourselves with a toolkit of techniques refined through experience to maneuver the complex terrain of algorithmic optimization with grace and wisdom as we uncover the subtleties of each method through case studies.
12. Conclusion
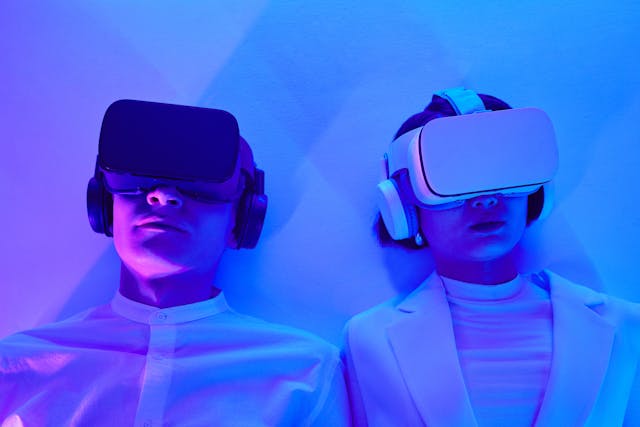
In conclusion, it is imperative to comprehend the differences between Divide and Conquer and Dynamic Programming. Divide and Conquer is a problem-solving technique that divides a problem into smaller, independent sections, solves each one separately, and then combines the results. Both strategies break down difficulties into smaller problems for solving. In contrast, Dynamic Programming builds on the solution of smaller problems by storing answers to subproblems in a table, hence reducing the need for duplicate computations.
The way these methods handle overlapping subproblems is a crucial point of divergence; Divide and Conquer does not save previous solutions, which results in the computation of the same subproblems repeatedly. On the other hand, the memorizing method of Dynamic Programming stores these outcomes for effective problem resolution.
Gaining proficiency with these algorithms is necessary for effective problem-solving in a variety of computational scenarios. Knowing whether to use dynamic programming over divide and conquer will have a big impact on how well your solutions work and how scalable they can be. By honing this ability, you'll have the tools you need to solve challenging issues and improve your algorithms.