1. Introduction to Web Scraping
One method for obtaining data from webpages is web scraping. It entails sifting through a webpage's HTML structure to extract particular data. This procedure can be helpful for gathering data for analysis or visualization, competitive analysis, and market research, among other things.
We will look at utilizing Node.js to create a web scraper in this blog post series. With the help of the robust JavaScript runtime Node.js, we can build scalable and effective server-side apps. With Node.js and well-known frameworks like Cheerio and Puppeteer, we can create powerful web scraping applications.
Join us as we explore the principles of web scraping using Node.js, examine several techniques for obtaining data from websites, tackle obstacles like dynamic content loading and anti-scraping methods, and finally construct our own web scraper from the ground up.
2. Setting Up NodeJS Environment
Creating an effective web scraper starts with configuring the Node.js environment. Because Node.js is known for its seamless handling of asynchronous operations, it is the perfect choice for web scraping tasks that call for concurrent processing. First things first, make sure Node.js is installed on your computer. You may do this by downloading and installing it from the official website or, for more flexibility in handling different Node.js versions, by using a version manager like NVM.
Starting a new Node project is an essential step after configuring Node.js. To accomplish this, make a new directory for your project and use the terminal to type `npm init}. To configure your project's name, version, description, entry point, test command, repository data, author information, license, and other details, simply follow the prompts.
After using npm to setup your project, it's time to install the necessary web scraping packages. `axios` is a widely used Node.js package for web scraping, which makes it easier to make HTTP requests and retrieve data from websites. Run `npm install axios} in your project directory to install `axios` using npm. Additional programs such as `cheerio} for data extraction from HTML and `puppeteer` for managing pages rendered with JavaScript could be required.
You can now begin creating the Node.js web scraper code after installing the required dependencies. While collecting data from websites, keep in mind to treat errors with grace, neatly arrange your code, and abide by their terms of service. The subsequent section of this tutorial series will cover building code to efficiently scrape a website and extract valuable data utilizing Node.js's features.
3. Installing Necessary Packages for Web Scraping
We will concentrate on installing the required packages for web scraping in this section of our tutorial on creating a web scraper using Node.js. Prior to beginning the process of extracting data from websites, we must equip our development environment with the necessary resources.💡
A vital component for Node.js web scraping is the `axios` package, which is used to send HTTP queries. With the help of this program, we may retrieve HTML content from websites and extract the necessary data. You may use the Node.js package manager npm to install {axios} by typing the following command into your terminal:
```bash
npm install axios
```
`cheerio` is another essential program for web scraping. Cheerio is a server-specific implementation of core jQuery that is quick, adaptable, and lean. It offers an easy-to-use API for navigating and working with HTML texts. npm can also be used to install {cheerio}:
```bash
npm install cheerio
```
You may want to think about utilizing `node-fetch` to handle parsing cookies and managing sessions while you're scraping websites. This package makes it easier to make HTTP requests by bringing window.fetch to Node.js. You can use the following npm command to install `node-fetch`:
```bash🗜
npm install node-fetch
```🎛
Lastly, you might need a headless browser like Puppeteer when working with websites that involve JavaScript rendering, such as those created using frameworks like React or Angular. Using the DevTools Protocol, a Node library called Puppeteer offers a high-level API for controlling Chrome or Chromium. Puppeteer may be installed with npm:
```bash
npm install puppeteer
```
Installing `axios`, `cheerio`, `node-fetch`, and Puppeteer` will provide you with a strong base upon which to launch your Node.js web scraping project. We will explore how to utilize these tools to properly and efficiently harvest data from websites in the future section of our series.
Stay tuned for more insights on how to build powerful web scrapers using Node.js!💬
4. Understanding the Basics of HTML/CSS Selectors
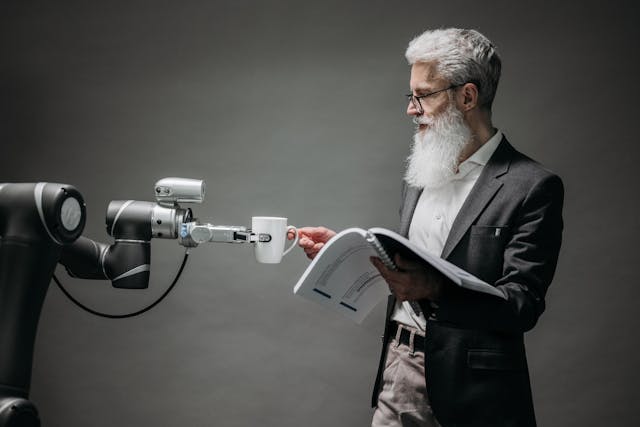
It is essential to comprehend HTML/CSS selectors when constructing a web scraper using NodeJS. Selectors assist in locating components on a webpage from which data extraction is desired. They function by concentrating on particular HTML components according to their attributes (id, class, tag name, etc.).
CSS selectors are helpful for web scraping in addition to being used to style online pages. To find the precise data you require, you can utilize CSS selectors like as class names or IDs. For instance, you might use a selector like ".product-name" to target all the components with that class if you wanted to retrieve product names from an online store.
An important factor in effectively choosing items is the HTML structure. Creating accurate CSS selectors requires an understanding of the hierarchy and nesting relationships between elements. When incorporating components and selectors into your scraping script, tools such as Chrome DevTools can help with the inspection and testing process.
Gaining proficiency with HTML/CSS selectors will enable your web scraper to efficiently browse webpages and reliably collect pertinent data. This fundamental understanding creates countless opportunities for efficiently obtaining important information from the internet.
5. Building a Simple Web Scraper in NodeJS
We will use NodeJS to create a basic web scraper in this part. The practice of mechanically obtaining data from websites is known as web scraping. We may take advantage of NodeJS's asynchronous features and libraries to simplify and expedite this procedure.
Installing a few prerequisites is the first step in setting up our web scraper. `cheerio} is a well-liked package in the NodeJS ecosystem for web scraping, including jQuery-like functionality for simple HTML parsing. Additionally, we can utilize `axios` to make HTTP queries in order to retrieve the content of the website that we wish to scrape.
Next, we'll create a function that retrieves a webpage's HTML content using Axios. After the HTML content is ready, we can load it into Cheerio and utilize its selector syntax to pull out particular information from the website. For instance, we can use Cheerio to extract the text from the relevant HTML elements and target them if we want to scrape every article title on a blog.💡
Using Cheerio, we may retrieve the appropriate data and then store or change it as needed. This can entail processing information further in our application, storing it to a file, or adding it to a database. Not only is it a useful skill to have, but building a basic NodeJS web scraper also opens up opportunities for effectively automating data collection activities.
6. Handling Errors and Edge Cases in Web Scraping
Taking error and edge case handling into consideration is essential when developing a web scraper with Node.js. Interacting with other websites when web scraping may result in unforeseen problems like network faults, timeouts, or structural changes to the website. It is imperative to integrate error handling procedures in order to guarantee the smooth and reliable operation of the scraper.
Try-catch blocks are a popular way to handle problems in web scraping. Any mistakes that arise during execution can be captured in the catch block by enclosing the scraping logic inside a try block. This enables you to manage exceptions politely by recording mistakes, attempting unsuccessful queries again, or ignoring troublesome URLs without bringing down the scraper as a whole.😽
Strong error-handling techniques, like exponential backoff retries, can be used to lessen transitory problems like rate limitation or brief network outages. You can increase the likelihood of successful data extraction while taking into account the server load of the website and the effectiveness of your scraper by gradually extending the duration between retries.
Anticipating and adjusting to changes in website structures is another factor to take into account while handling edge cases with web scraping. The frequent alterations made to websites could affect how accurately the scraper extracts data. To overcome this difficulty, keep an eye out for updates on the target websites and modify your scraping logic as necessary. 😬
You can improve your scraper's resistance to dynamic content and intricate website layouts by using technologies like headless browsers or specialized libraries that provide more sophisticated DOM parsing capabilities. These technologies can facilitate data extraction from JavaScript-rendered pages, get over anti-scraping measures, and traverse interactive components.
A proactive strategy that combines strong error handling mechanisms with ongoing monitoring and adaption to changes in target websites is needed to address mistakes and edge cases in web scraping. You can create a dependable and effective web scraper using Node.js that regularly produces correct results by foreseeing possible problems and putting relevant solutions in place in advance.
7. Advanced Techniques: Pagination and Dynamic Content Loading
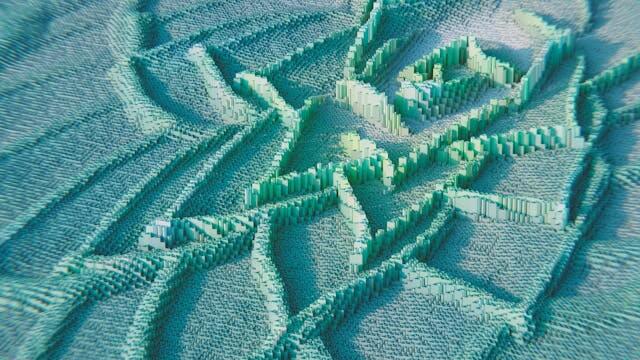
In this more complex portion of creating a NodeJS web scraper, we will look at methods for managing pagination and dynamic content loading. These are typical difficulties encountered when scraping webpages that load dynamically or have a lot of content dispersed over several pages.
It's critical to understand the website's page link structure when working with pagination. This could entail searching for particular HTML elements that point to the next page link or examining the URL parameters, which alter as you go through the sites. Once located, these URLs can be methodically iterated over by your scraper to retrieve data from each page.
Another problem with dynamic content loading is that a lot of contemporary websites load data asynchronously after the page loads using JavaScript. You can use programs like Puppeteer to mimic a browser environment and interact with the page as a user would in order to scrape dynamically loaded material. This enables you to retrieve the needed information after waiting for the dynamic material to load.
Through the integration of pagination and dynamic content loading mechanisms, a NodeJS web scraper allows you to safely and rapidly browse intricate websites while retrieving the data you require. These cutting-edge techniques improve your scraping skills so you can successfully take on increasingly difficult scraping assignments.
8. Writing Data to a File or Database
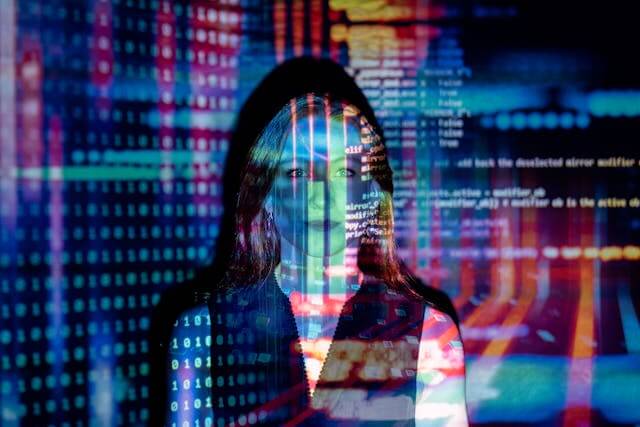
Keeping the data for later use is the next step after using your web scraper to collect the needed info. You may easily write this data to a file or database in NodeJS. One easy method is to use Node's fs module to create a JSON file and save the data in it.😃
Requiring the 'fs' module is the first step in using NodeJS to write data to a file. The data can then be written to a chosen file using the fs.writeFile method. The parameters for this function are the file name, the data to write, and a callback function that will be used when the writing is finished.
You will need to establish a connection between your NodeJS application and the database if you decide to save your scraped data in a MongoDB database. For MongoDB interaction, use programs like mongoose; for SQL databases, use Sequelize.
Working with JavaScript objects instead of raw queries makes working with databases easier when you use an ORM (Object-Relational Mapping) like Mongoose or Sequelize. After you're linked, you can preserve the data that was scraped by defining models that correspond to your data structure.
Later on, you may create more sophisticated queries and manipulate your data more easily if you store your scraped data in a database as opposed to just files. As your dataset expands over time, it also makes scaling your scraping job easier.
9. Best Practices in Web Scraping Ethics and Legal Considerations
Respecting legal and ethical boundaries is essential when participating in web scraping. Observe the robots.txt file and the terms of service on the website. Be careful not to overburden the servers of the target website with your scraping efforts. Always include a clear self-identification in the user-agent string to make your intentions transparent.
Steer clear of collecting private or copyrighted content or other sensitive information without authorization. Pay attention to the information you gather, treat it carefully, and abide by any applicable data protection laws, such as the CCPA and GDPR. When in doubt, get legal counsel to make sure that web scraping rules and regulations in your jurisdiction are followed.
Recall that, even while online scraping can be an effective method of obtaining information, it must be done in an ethical and responsible manner. You can use web scraping productively and respect other people's rights and privacy at the same time by adhering to best practices and thinking through the legal ramifications of your conduct.
10. Testing and Debugging Your Web Scraper
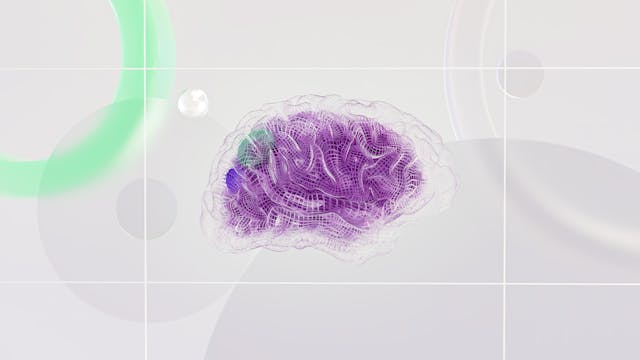
To make sure your web scraper is dependable and effective, it is essential to test and debug it. You may create test cases for your scraper in Node.js using a variety of testing frameworks, such as Mocha or Jest. These tests can assist in verifying that your scraper operates as intended in various situations and environments.
When creating tests for your web scraper, think about incorporating end-to-end testing to replicate actual use cases, integration testing to confirm interactions between various scraper components, and unit testing to examine individual components. This thorough testing procedure will keep your scraper codebase up to date and help identify vulnerabilities early.🖲
Use tools like the VS Code debugger or Chrome DevTools to debug your Node.js web scraper. You may analyze variables, set breakpoints, and track the execution path of your scraper code with these tools. Your web scraping application's accuracy and performance can be enhanced by methodically debugging and finding and resolving problems.
11. Scaling Up Your Web Scraper for Larger Projects
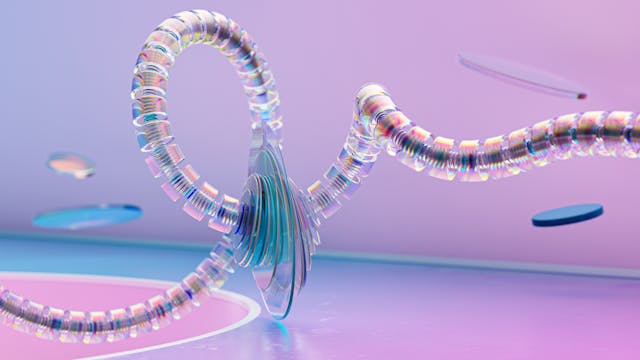
To make sure your web scraper can handle more data successfully and efficiently, you must scale it up for larger projects. Increasing the performance of your code is one approach to do this. Think about providing suitable error handling and retry methods, optimizing asynchronous operations for quicker processing speed, and rewriting your code to make it more modular and reusable.
If you want to prevent overloading a single machine, you might need to think about splitting the workload over several instances or servers. This can be achieved by managing the allocation of jobs among various workers by putting up a task queue system, such as RabbitMQ or Redis queue. By enabling you to run functions concurrently, cloud platforms such as AWS Lambda or Google Cloud Functions can further aid in scaling your scraper.
Resource management is another crucial component of growing your web scraper. To prevent being blocked or banned, be careful to keep an eye on and modify your frequency of scraping in accordance with the terms of service and rate limits of the website. When working with massive datasets, putting caching methods in place can also assist cut down on pointless requests and enhance overall speed.
For bigger projects, scaling up a web scraper necessitates meticulous planning, code optimization, effective resource management, and sometimes the use of distributed computing methods. By using these techniques, you can create a scalable and reliable web scraper that can easily handle even the trickiest scraping jobs.